Operators in JavaScript are special symbols used to perform operations over operands (Values and Variables).
Example:
// Example of operator 2 + 3 = 5; // Here + and = both are operators
JavaScript Operator Types
- Arithmetic operators
- Assignment operators
- Comparison operators
- Logical operators
- Bitwise operators
Let’s discuss one by one below
1. Arithmetic Operators in Js
Arithmetic operators are used to perform arithmetic operations on operands.
For example:
const num = 6 + 3; // 9
Here, the +
operator is used to add two operands.
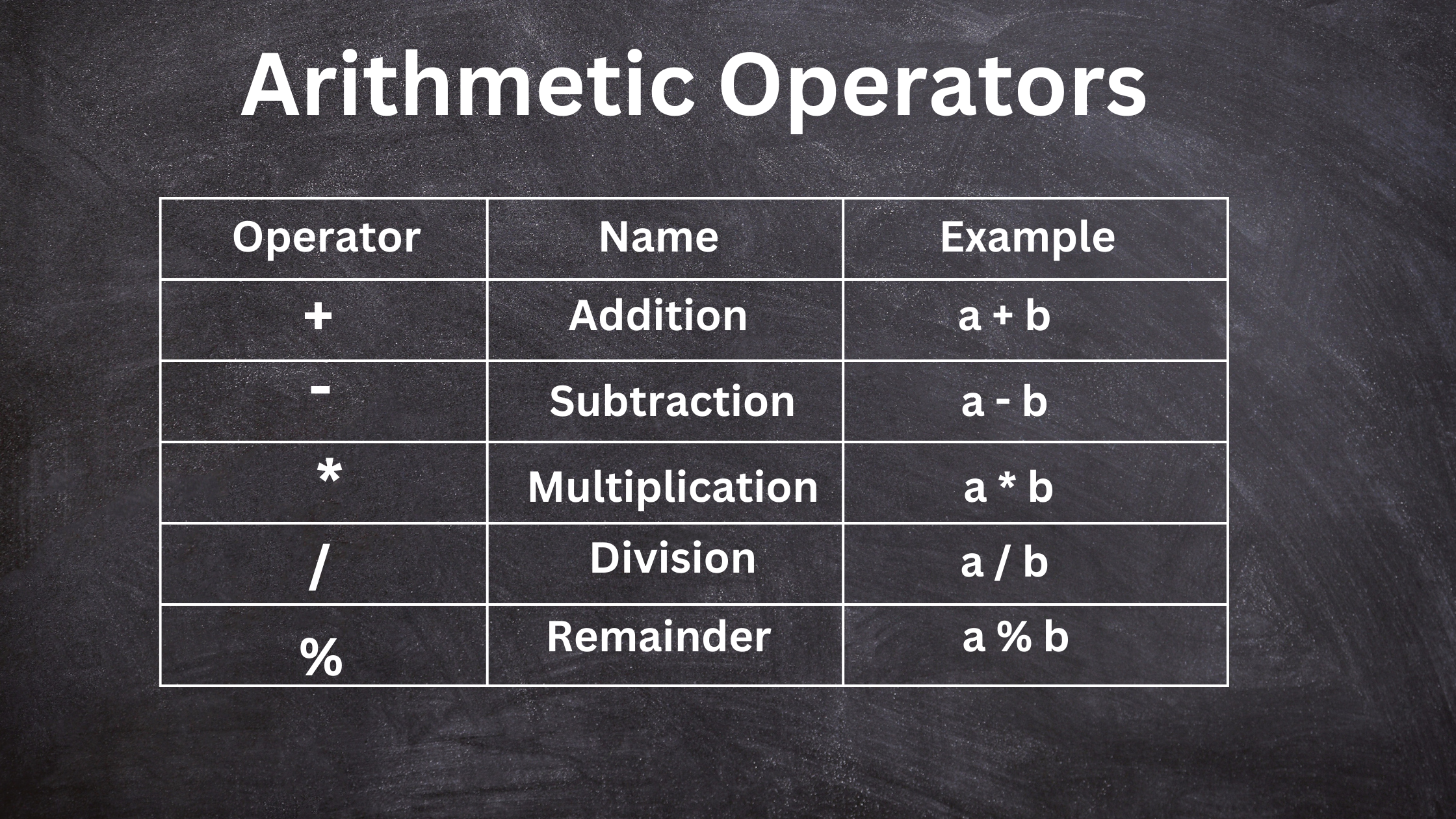
Example: Arithmetic Operators in JavaScript
// Example of Arithmetic Operators let a = 5; let b = 2; // addition operator console.log('a + b = ', a+b); // 7 // subtraction operator console.log('a - b = ', a - b); // 3 // multiplication operator console.log('a * b = ', a * b); // 10 // division operator console.log('a / b = ', a / b); // 2.5 // remainder operator console.log('a % b = ', a % b); // 1
2. Assignment Operators
Assignment operators are used to assigning values to variables.
For example:
const a = 5; // Here 5 is assigned to variable a
Here, the =
operator is used to assign the value 5 to the variable a.
Here is a list of the most commonly used assignment operators:
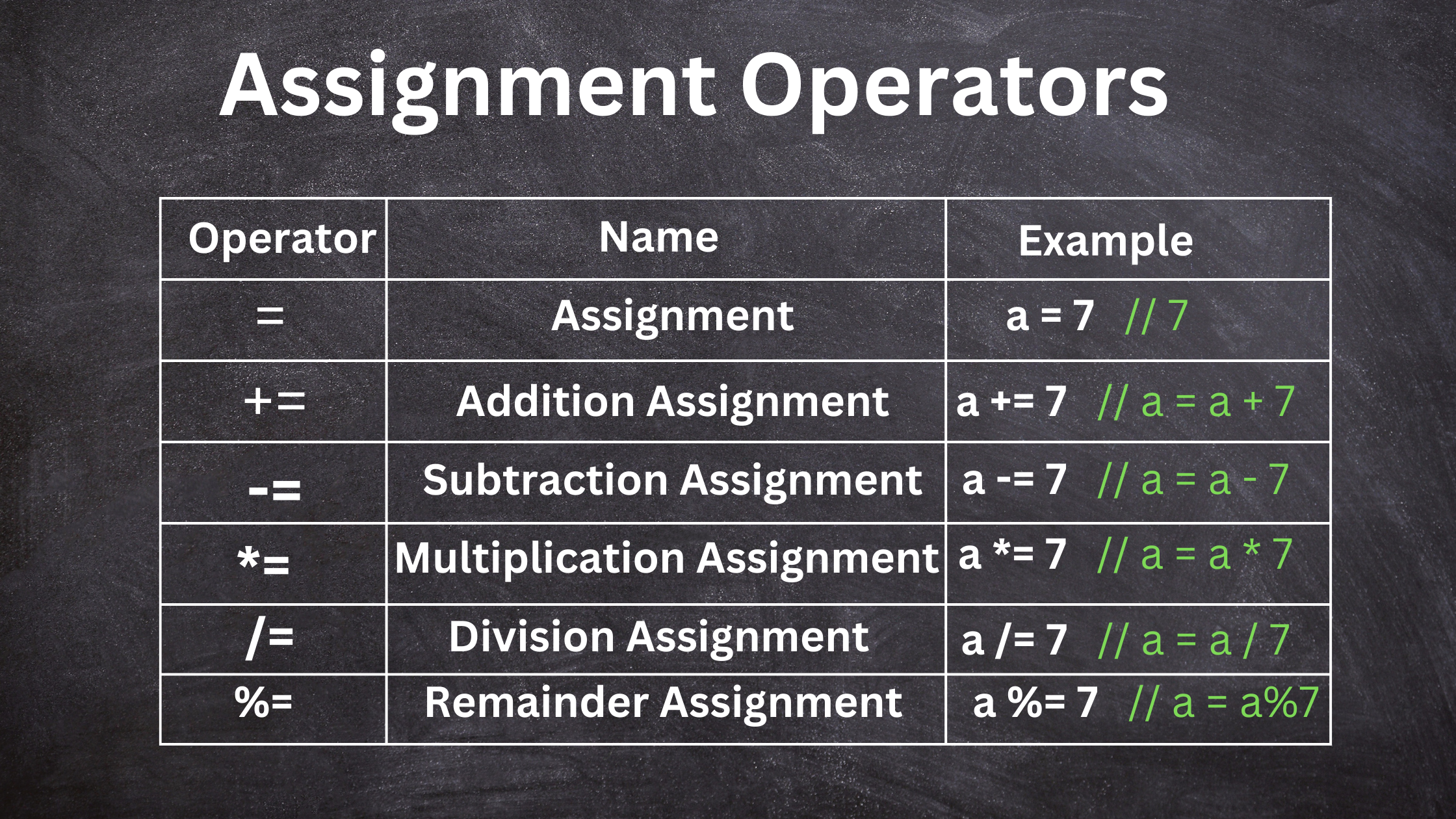
3. Comparision Operators
The comparison operators compare two values and return a boolean value, either true or false .
For example:
// Example of Comparision Operator const a = 5, b = 1; console.log(a > b); // true
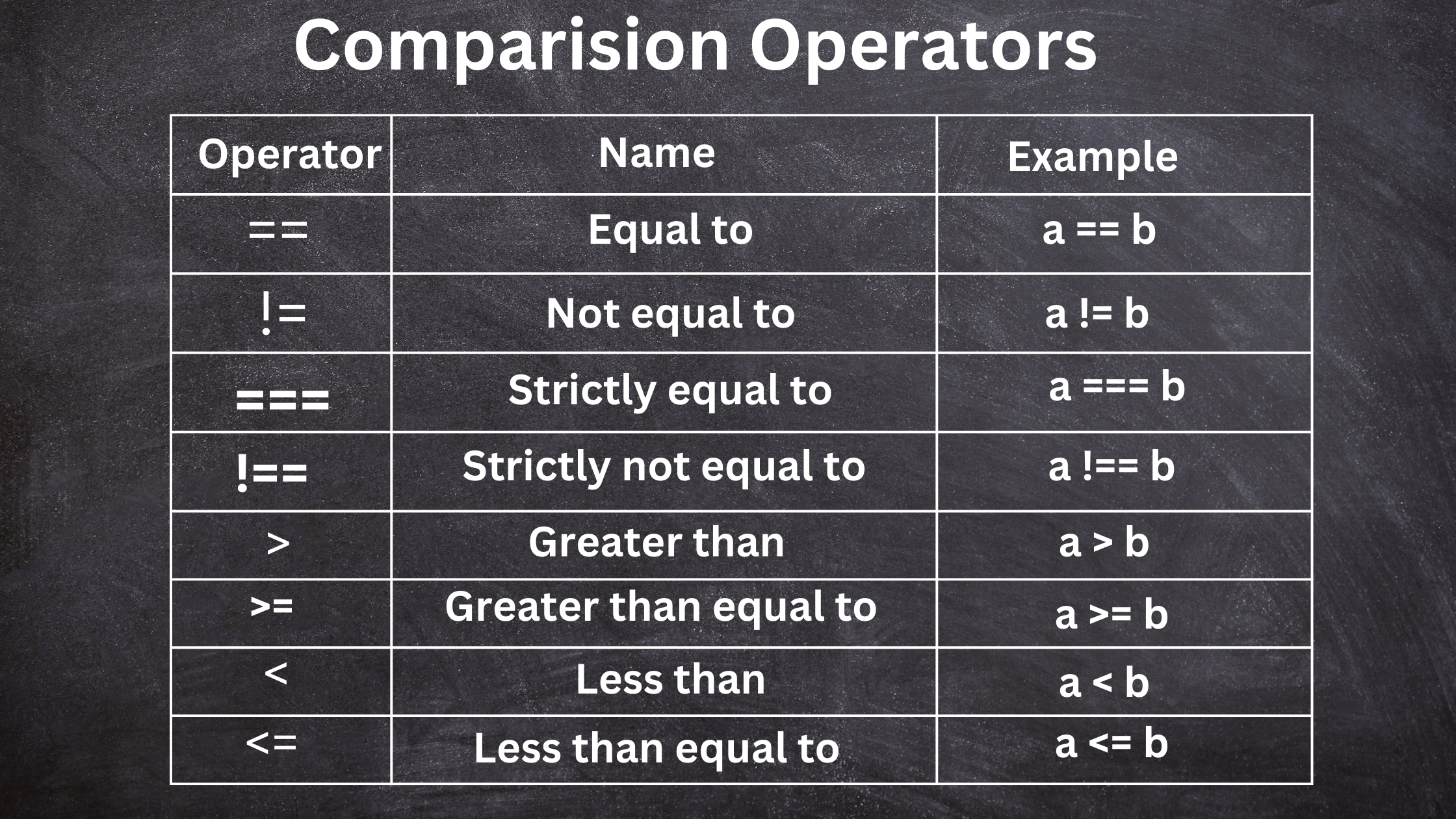
Example: Comparision Operators in JavaScript
// Comparision Operators // Equal operator console.log(5 == 5); // true console.log(5 == '5'); // true // Not Equal operator console.log(6 != 4); // true console.log('hey' != 'Hey'); // true // Strict Equal operator console.log(5 === 5); // true console.log(5 === '5'); // false // Strict Not Equal operator console.log(5 !== '5'); // true console.log(5 !== 5); // false
Comparision or Relational operators are used in decision making and in loops. You will learn more about the use of comparison operators in later articles.
4. Logical Operators
Logical operators perform logical operations and return a boolean value, either true or false.
Example:
//Example of Logical Operator const a = 5, b = 3; (x < 8) && (y < 10); // true
Here && is the logical operator AND. Since a < 8 and b < 10 are true, the result is true.
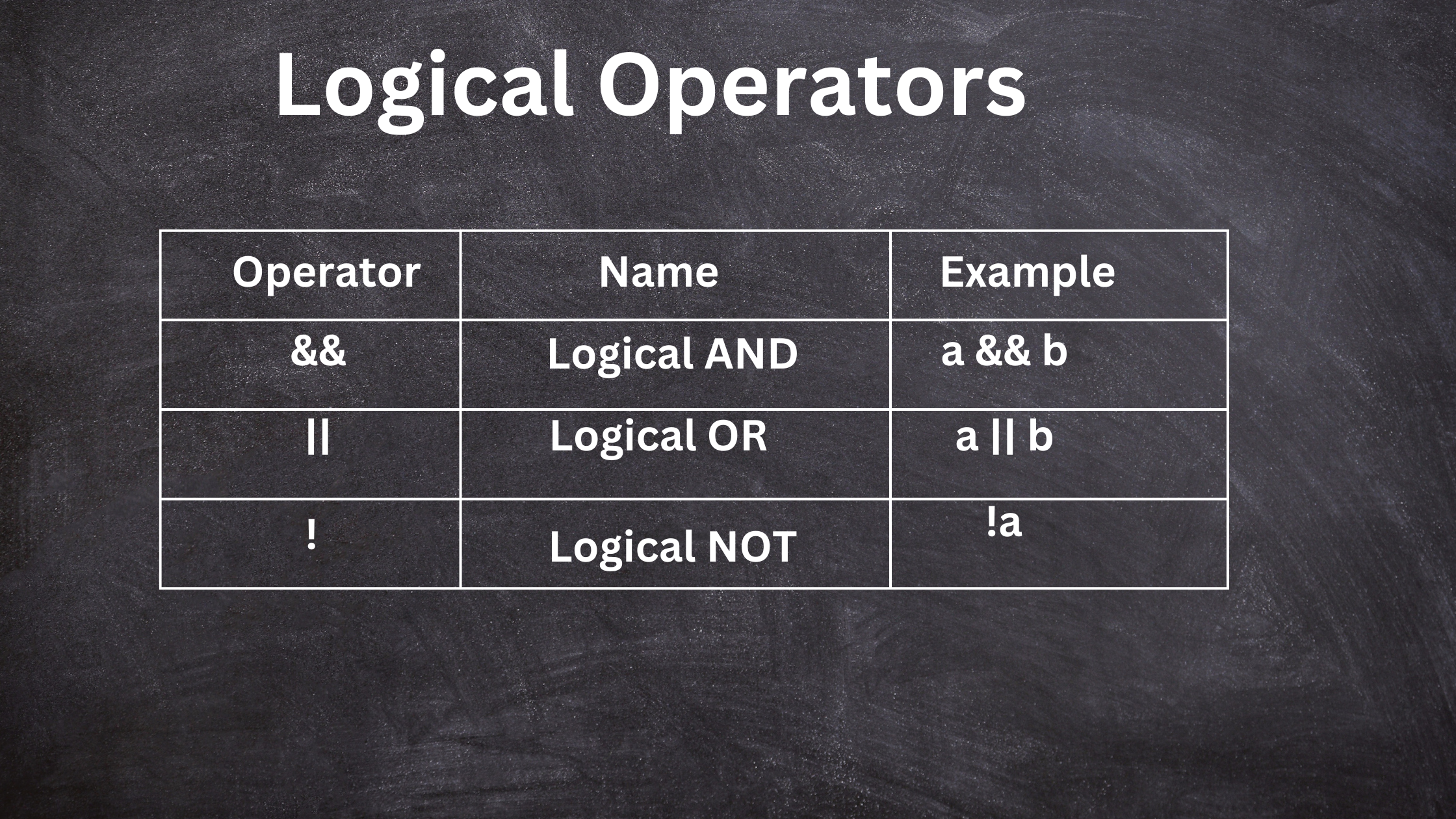
Example: Logical Operators in JavaScript
// Logical AND console.log(true && true); // true console.log(true && false); // false // Logical OR console.log(true || false); // true // Logical NOT console.log(!true); // false
Logical operators are used in decision making and in loops. You will learn more about using logical operators in detail in later articles.
5.Bitwise Operators
Bitwise operators are used to perform operations on binary representations of numbers.
For example:
// Example of bitwise operator let x = 11; let y = 21; result = x & y; console.log(result); // 1
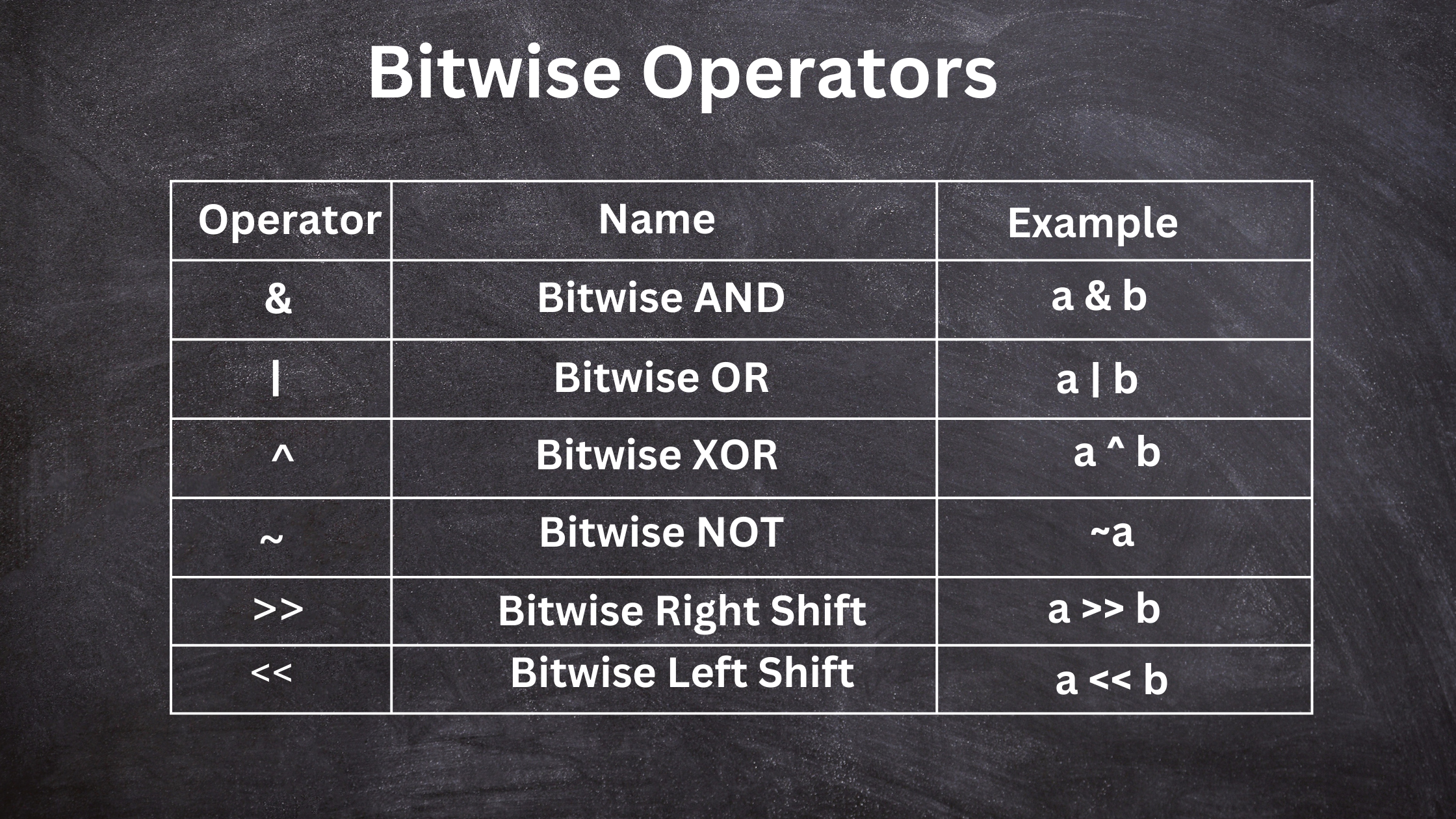
Bitwise operators are rarely used in day-to-day programming. If interested, please visit JavaScript Bitwise Operators for more information.
Thank you so much for reading
Happy Coding
Some more Articles on JavaScript